문제 발생
node.js 와 React에서 Socket 통신 시
GET http://localhost:3010/socket.io/?EIO=4&transport=polling&t=NezM72w 404 (Not Found
위와 같은 에러가 발생했다.
아래는 문제의 코드이다.
app.ts
//app.ts
import express from "express";
import cors from "cors";
import http from "http";
import webSocket from "./socket";
const app = express();
app.use(cors());
const PORT = 3010;
const httpServer = http.createServer(app);
webSocket(httpServer);
httpServer.listen(PORT, () => console.log(`app listening on port ${PORT}!`));
socket.ts
import http from "http";
import sortConverter from "./util/sortConverter";
import { Server } from "socket.io";
let interval: number = 3000;
export default function webSocket(httpServer: http.Server) {
const io = new Server(httpServer);
io.on("connection", (socket) => {
console.log("New client connected");
socket.on("disconnect", () => console.log("user disconnect", socket.id));
socket.on("reply", (data) => {
console.log(data);
});
setInterval(() => {
socket.emit("FromAPI", sortConverter());
}, interval);
});
}
아직 node.js에 익숙하지 않은 나는 위의 코드에서 잘못된 점을 찾지 못하고 헤매었다..
해결 과정
위의 코드를 위에서부터 한 줄씩 읽어 내려왔다.
import express from "express";
import cors from "cors";
import http from "http";
import webSocket from "./socket";
const app = express();
app.use(cors());
const PORT = 3010;
const httpServer = http.createServer(app); //http 기반의 server 생성, 요청이 들어올 때마다 콜백함수 실행
webSocket(httpServer); //socketIo에 server 개체를 전달
app.listen(PORT, () => console.log(`app listening on port ${PORT}!`)); // 접속하는 port를 할당하기 위한 listen 함수
해결
express 공식문서에서 http.createServer()에 대한 내용을 확인하면 아래와 같은 문구가 있다.
- http 모듈을 이용해 직접 작업해야 하는 경우(socket.io/SPDY/HTTPS)를 제외하면, http 모듈이 더 이상 필요하지 않습니다. app.listen() 함수를 이용해 앱을 시작할 수 있습니다.
const PORT = 3010;
const httpServer = http.createServer(app);
webSocket(httpServer);
app.listen(PORT, () => console.log(`app listening on port ${PORT}!`));
나는 socket.io를 사용하면서 app.listen() 함수를 사용해서 웹소켓이 정상적으로 작동되지 않았다는 것을 알게 됐다.
httpServer.listen(PORT, () => console.log(`app listening on port ${PORT}!`));
위와 같이 수정해주니 통신이 된다.
그런데 통신은 되었지만 CORS 에러가 등장했다.
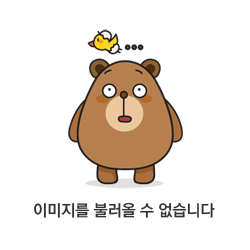
app.use(cors()); //server에 할당 해주어야 함
위와 같이 cors 처리를 해주었지만 socket은 socket.Io모듈에 별도로 지정해 주어야 한다고 한다.
//socket.ts
import http from "http";
import sortConverter from "./util/sortConverter";
import { Server } from "socket.io";
let interval: number = 3000;
export default function webSocket(httpServer: http.Server) {
const io = new Server(httpServer, {
cors: {
origin: "*",
},
});
io.on("connection", (socket) => {
console.log("New client connected");
socket.on("disconnect", () => console.log("user disconnect", socket.id));
socket.on("reply", (data) => {
console.log(data);
});
setInterval(() => {
socket.emit("FromAPI", sortConverter());
}, interval);
});
}
위의 코드에서 아래의 부분을 추가하면 된다.
export default function webSocket(httpServer: http.Server) {
const io = new Server(httpServer, {
cors: {
origin: "*",
},
});
'node.js' 카테고리의 다른 글
[Node.js] grpc 통신 WebSocket POST Request 방법 (0) | 2022.03.08 |
---|---|
[node.js] node 최신 버전 업그레이드 및 버전 변경 (nvm) (0) | 2021.12.31 |
[Node.js] Stack trace (스택 추적) (0) | 2021.09.08 |
[Node.js] Node js + TypeScrpt + ESLint(airbnb) + Prettier 적용 (2) | 2021.07.08 |
(node.js + React) + Socket.io 간단한 연결 구현 (+ TypeScript) (0) | 2021.06.25 |